OpenAPI Extensions
We've added a few OpenAPI extensions to help you better integrate with ReadMe.
Getting Started
Extensions are properties added to an OpenAPI spec that customize your API Reference user experience. All of these properties are optional.
ReadMe extensions are defined by the x-readme
object and most can be placed either on the root level of your spec, or on the operation level. See the Usage section below for specific details.
Want to see a spec file that utilizes these extensions? Take a look at the example demo spec file here.
Usage
Unless otherwise noted below all of our custom OpenAPI extensions can be defined on the root level of your API definition within a x-readme
object:
{
"openapi": "3.0.2",
"info": {
"title": "Owl Parliament",
"description": "This is a sample Owl Parliament Server",
"version": "1.0.0"
},
"x-readme": {
"explorer-enabled": false,
"proxy-enabled": false,
"samples-languages": ["shell", "python", "go"]
}
}
If you don't want to use x-readme
in your spec, you can define each extension as x-<extension_name>
instead:
{
"openapi": "3.0.2",
"info": {
"title": "Owl Parliament",
"description": "This is a sample Owl Parliament Server",
"version": "1.0.0"
},
"x-explorer-enabled": false,
"x-proxy-enabled": false,
"x-samples-languages": ["shell", "python", "go"]
}
Operation-level usage
You can also define each extension on the operation-level to individually control their functionality on an operation-by-operation basis. If an extension is defined at both the operation and root levels, the operation-level extension takes presedence.
{
"paths": {
"/owl": {
"post": {
"summary": "Add a new owl to the parliament",
"description": "Adds a new owl to the parliament",
"operationId": "addOwl",
"x-readme": {
"samples-languages": ["shell", "python", "go"]
}
}
}
}
}
You can also define the extension using the x-<extension_name>
format instead:
{
"paths": {
"/owl": {
"post": {
"summary": "Add a new owl to the parliament",
"description": "Adds a new owl to the parliament",
"operationId": "addOwl",
"x-proxy-enabled": false,
"x-samples-languages": ["shell", "python", "go"]
}
}
}
}
Available Extensions
Disable the API Explorer
Disables the API Explorer's "Try It" button, preventing users from making API requests from within your docs. Users will still be able to fill out any entry fields (path or query parameters, etc.), and code snippets will be auto-generated based on the user's input, however to interact with your API the user will need to copy the code snippet and execute it outside of your docs.
This does not disable your API Reference documentation.
Extension | Default value |
---|---|
x-readme.explorer-enabled | true |
{
"x-readme": {
"explorer-enabled": true
}
}
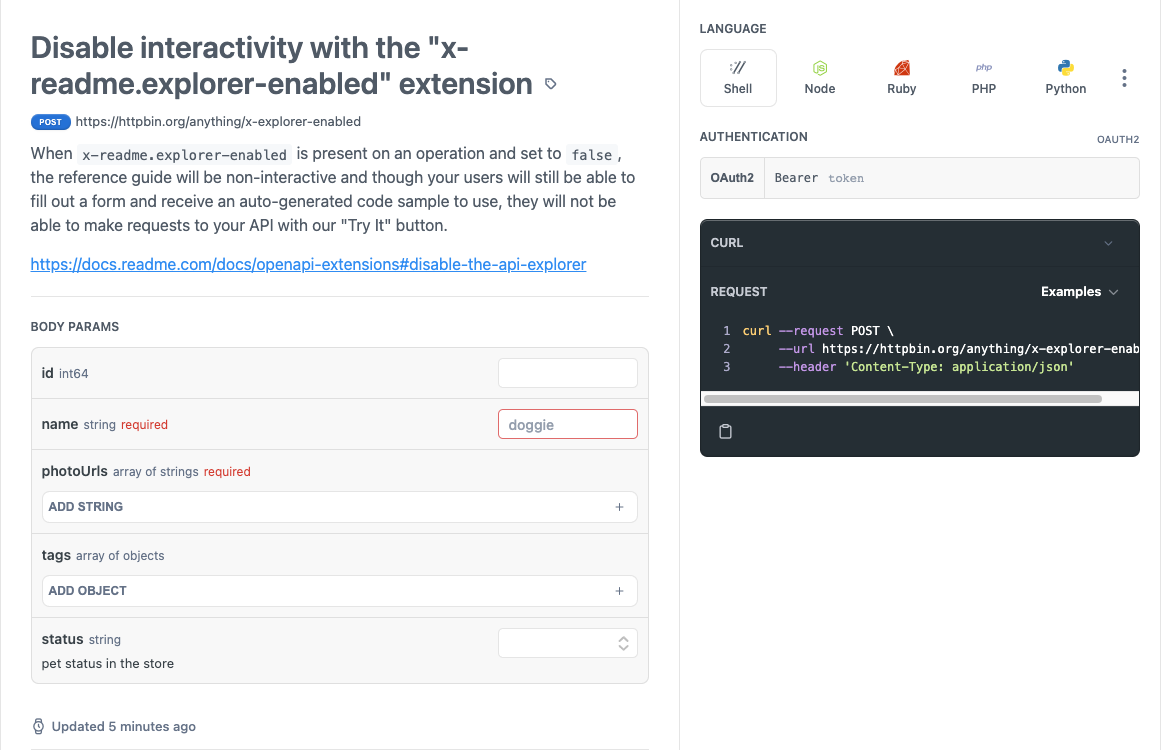
Screenshot of the API Explorer with the "Try It" button disabled and omitted. Note that filled out fields will still update the code sample.
Code sample languages
Toggles what languages are shown by default for code samples in the API Explorer. This only affects what languages are initially shown to the user; if the user picks another language from the three-dot menu, that language and the respective auto-generated code snippet will also appear as an option in the API Explorer.
We built a Node package called
api
that magically generates an SDK from an OpenAPI definition! To seeapi
in action visit our API documentation!
Extension | Default value |
---|---|
x-readme.samples-languages | ['shell', 'node', 'ruby', 'javascript', 'python'] |
{
"x-readme": {
"samples-languages": ["shell", "node", "ruby", "javascript", "python"]
}
}
These are the languages supported by way of the httpsnippet library:
Language | Available language mode(s) | Libraries (if applicable)* |
---|---|---|
C | c | Libcurl |
Clojure | clojure | clj-http |
C++ | cplusplus | Libcurl |
C# | csharp | HttpClient, RestSharp |
HTTP | http | HTTP/1.1 |
Go | go | NewRequest |
Java | java | AsyncHttp, java.net.http, OkHttp, Unirest |
JavaScript | javascript | XMLHttpRequest, Axios, fetch, jQuery |
JSON | json | Native JSON |
Kotlin | kotlin | OkHttp |
Node.js | node | api , HTTP, Request, Unirest, Axios, Fetch |
Objective-C | objectivec | NSURLSession |
OCaml | ocaml | CoHTTP |
PHP | php | cURL, Guzzle, HTTP v1, HTTP v2 |
Powershell | powershell | Invoke-WebRequest, Invoke-RestMethod |
Python | python | Requests |
R | r | httr |
Ruby | ruby | net::http |
Shell | shell | cURL, HTTPie, Wget |
Swift | swift | URLSession |
*This column documents any supported HTTP libraries for a given language mode. The x-readme.samples-languages
array should contain language values (i.e. values from the second column like node
or php
) and not library names (i.e., values from the third column like axios
or guzzle
).
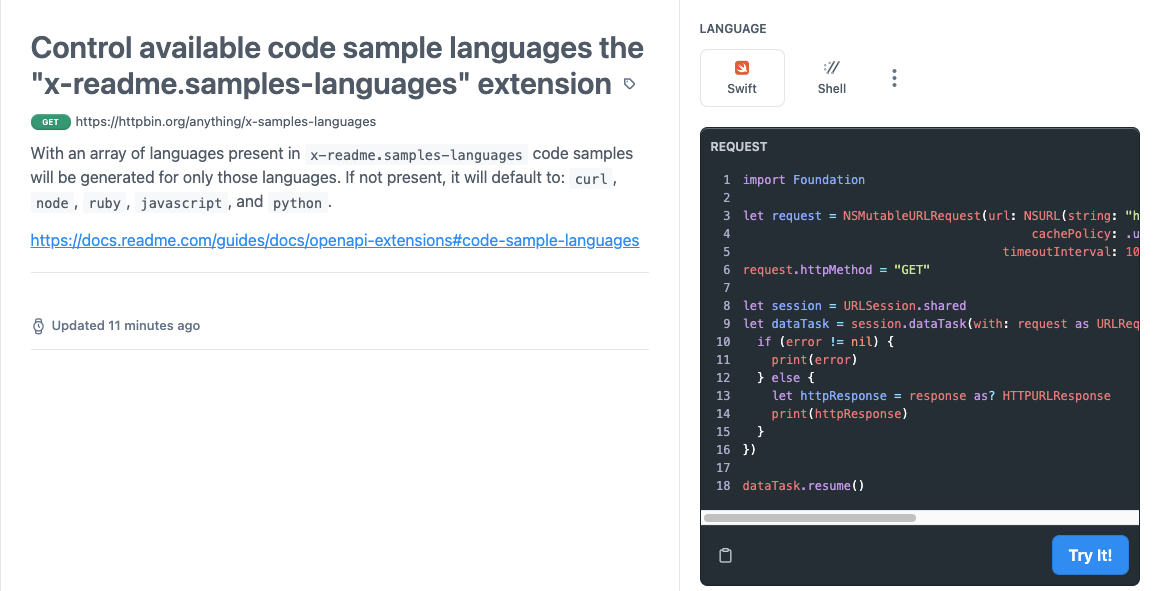
Screenshot of the API Explorer with "x-readme.sample-languages" set to Swift; note the Swift language shows up first above the code snippet.
Custom code samples
Enables custom-written code samples to be set for your operations. Use this if you have specific formatting that may not be followed by the auto-generated code samples.
Custom code samples are treated as static content, so it's not possible to populate a code sample with enduser data from a custom login (JWT).
This extension can only be set on the operation level
Extension | Default value |
---|---|
x-readme.code-samples | [] |
This must be provided as an array of JSON objects containing:
Key | Requirement | Description |
---|---|---|
language | Required | The language of the code sample. See the list of supported languages above. |
code | Required | Your custom code. It should preferrably be neat and tidy. |
name | Optional | A short name for your code sample. If none is provided we use the creative name default . |
install | Optional | If an external library is required for your code sample to function this can be a single command your users can run to install that library. For example of your code sample requires a custom Ruby SDK you can set this as gem install our-inhouse-sdk . |
correspondingExamples | Optional | If a response example should be displayed alongside this code sample, specify the response example's unique identifier using this field. When users select this code sample, the corresponding response example will automatically be selected. |
"x-readme": {
"code-samples": [
{
"language": "curl",
"code": "curl -X POST https://api.example.com/v2/alert",
"name": "Custom cURL snippet",
"install": "brew install curl",
"correspondingExample": "TestExample"
},
{
"language": "php",
"code": "<?php echo \"This is our custom PHP code snippet.\"; ?>",
"name": "Custom PHP snippet"
}
]
}
Corresponding Response Examples
Note how the correspondingExample
value in the above definition is TestExample
and the Responses Object below contains that TestExample
response example. With this setup, the response example below is automatically selected when a user selects that first cURL code sample above. In order to use this feature, you must use the examples
map approach to defining examples.
responses:
'200':
description: OK
content:
application/json:
examples:
TestExample: # the identifier is on this line!
summary: An example of a cat
value:
name: Fluffy
petType: Cat
color: White
gender: male
breed: Persian
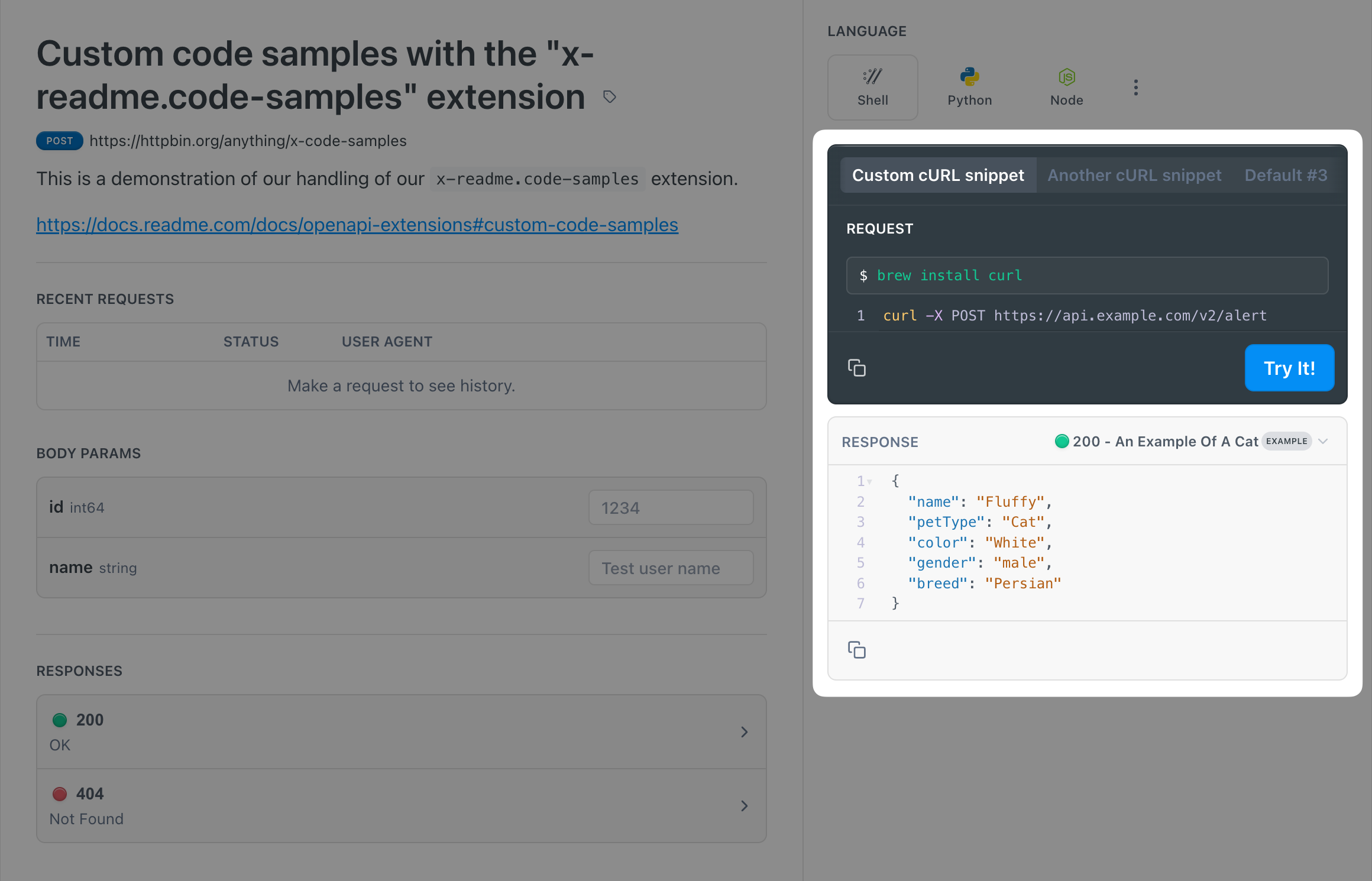
Screenshot of the API Explorer with an example spec utilizing the x-code-samples extension. Note the tabs showing different snippets for the cURL (Shell) language.
Enum Descriptions Table
Generates a table so descriptions can be added for each enum option. This will need to placed at the schema level where the enum is defined.
This extension may look familiar for folks coming from Redocly! Because this extension is used by other vendors, it should not be contained within an
x-readme
object and instead should live at the schema level.
Extension | Default value |
---|---|
x-enumDescriptions | {} |
{
"type": "string",
"enum": ["Owlbert", "Lorem Ipsum"],
"x-enumDescriptions": {
"Owlbert": "ReadMe's mascot",
"Lorem Ipsum": "dolor sit amet"
}
}
CORS proxy enabled
Toggles the CORS proxy used when making API requests from within your docs (via the "Try It" button). If your API is already set up to return CORS headers, you can safely disable this feature.
Disabling the CORS proxy will make the request directly from the user's browser and will prevent Metrics data from being logged by us unless Metrics have already set up on your backend.
Extension | Default value |
---|---|
x-readme.proxy-enabled | true |
{
"x-readme": {
"proxy-enabled": true
}
}
Parameter ordering
Controls the order of parameters on your API Reference pages.
Your custom ordering must contain all of our available parameter types:
path
: Path parametersquery
: Query parametersbody
: Non-application/x-www-form-urlencoded
request body payloadscookie
: Cookie parametersform
:application/x-www-form-urlencoded
request body payloadsheader
: Header parameters
Extension | Default value |
---|---|
x-readme.parameter-ordering | ['path', 'query', 'body', 'cookie', 'form', 'header'] |
{
"x-readme": {
"parameter-ordering": ["path", "query", "header", "cookie", "body", "form"]
}
}
Static headers
Adds static headers to add to each request. Use this when there are specific headers unique to your API.
Extension | Default value |
---|---|
x-readme.headers | [] |
Headers must be provided as an array of objects with key
and value
properties.
{
"x-readme": {
"headers": [
{
"key": "X-Static-Header-One",
"value": "owlbert"
},
{
"key": "X-Static-Header-Two",
"value": "owlivia"
}
]
}
}
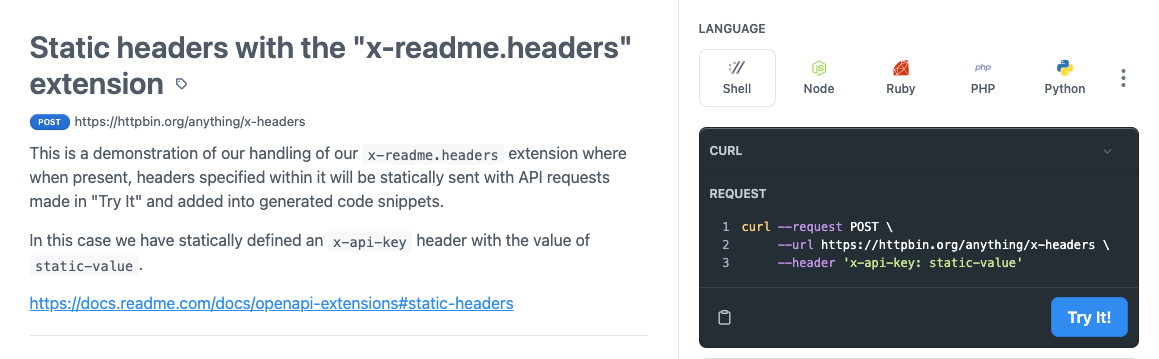
Screenshot of the API Explorer with a custom static header "x-api-key" defined in its spec.
Authentication defaults
Sets the default API Key or OAuth2 token used when making API requests from your docs. Other authentication types are not supported.
Unlike the other extensions we provide, authentication defaults are set using x-default
in your securitySchemes
object. Defaults can only be set for oauth2
and apiKey
authentication types.
{
"securitySchemes": {
"oauth": {
"type": "oauth2",
"x-default": "TXlOYW1lSXNPd2xiZXJ0IQ==",
"flows": {
"authorizationCode": {
"authorizationUrl": "https://example.com/oauth/authorize",
"tokenUrl": "https://example.com/oauth/token",
"scopes": {
"write:owls": "modify owls in your account",
"read:owls": "read your owls"
}
}
}
}
}
}
{
"securitySchemes": {
"type": "apiKey",
"name": "api_key",
"in": "header",
"description": "An API key that will be supplied in a named header.",
"x-default": "TXlOYW1lSXNPd2xiZXJ0IQ=="
}
}
Disable API Metrics
Disables API requests from the API Explorer's "Try It" button from being sent into our API Metrics for you and your users. Additionally on any API endpoint that this is disabled on your users will not see lists or graphs of previous requests they've made against that API endpoint β either through the API Explorer's interactivity or through one of our Metrics SDKs (if you have those installed on your API).
Extension | Default value |
---|---|
x-readme.metrics-enabled | true |
Deprecated and Unsupported Extensions
The extensions below are deprecated or are no longer supported in the most recent version of the API Reference Design. These are documented for reference purposes only.
Send defaults
This extension is deprecated and all defaults will be sent via the API Explorer.
Whether to send the defaults specified in your OpenAPI document, or render them as placeholders.
Extension | Default value |
---|---|
x-readme.send-defaults | false |
{
"x-readme": {
"send-defaults": false
}
}
Disable code examples
This extension is no longer supported.
Disable auto-generated code samples from appearing on your API Reference operation documentation.
Extension | Default value |
---|---|
x-readme.samples-enabled | true |
{
"x-readme": {
"samples-enabled": true
}
}
Support
Questions? Contact Support, we don't bite!
Updated 5 minutes ago